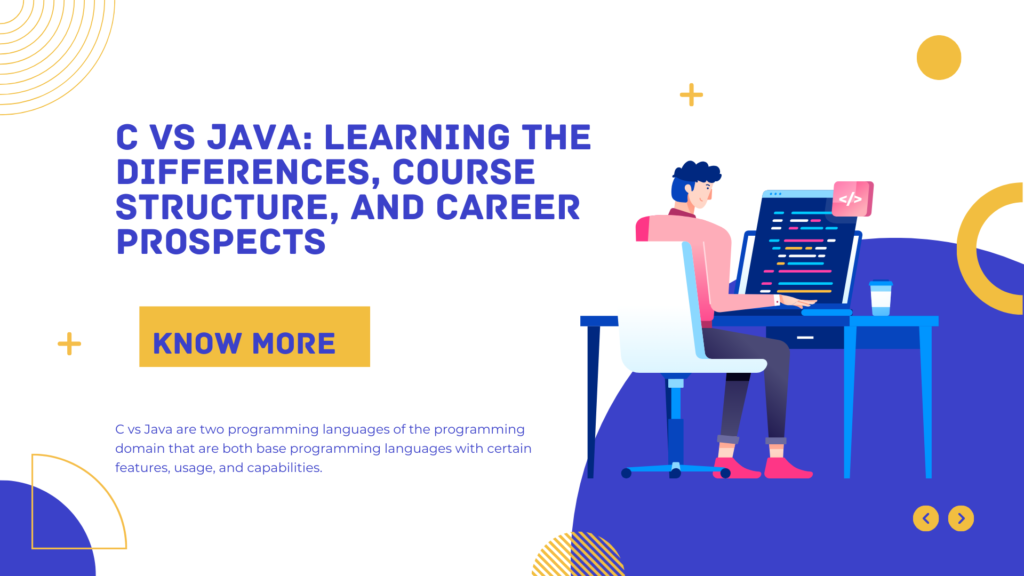
1. C and Java Overview
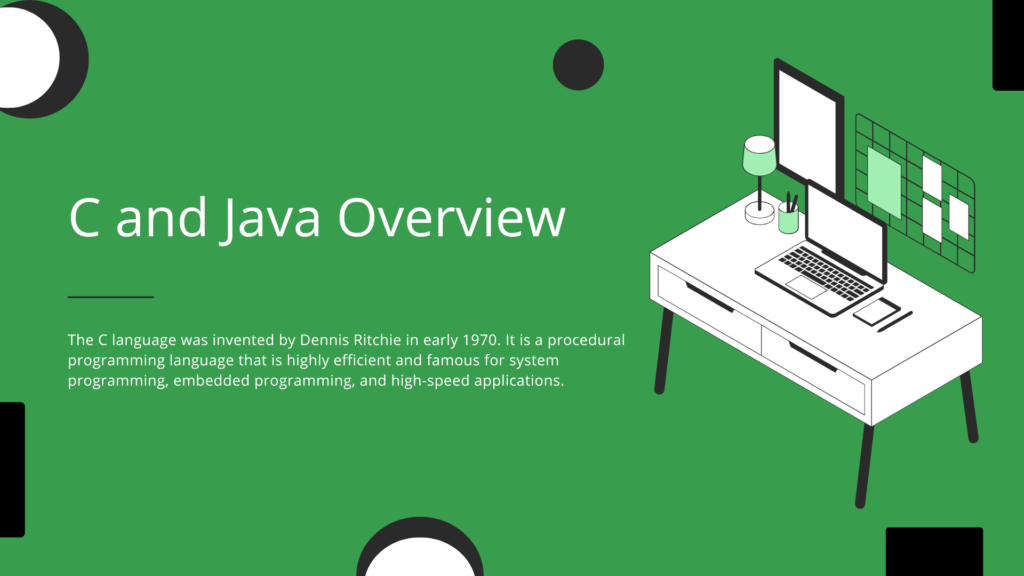
C Language:
The C language was invented by Dennis Ritchie in early 1970. It is a procedural programming language that is highly efficient and famous for system programming, embedded programming, and high-speed applications. It makes it possible to manage memory at a low level and, therefore, allows the hardware to be dealt with directly by the programmer.
Java Programming Language: Developed by James Gosling and Mike Sheridan in 1991 and subsequently sold to Sun Microsystems (now owned by Oracle). Java is an object-oriented programming (OOP) language that is independent of the platform. The fundamental concept of Java is “Write Once, Run Anywhere” (WORA), or the Java programs can be run on any platform where a Java Virtual Machine (JVM) is present.
2. Major Differences between C and Java
C: C possesses a very simple procedural syntax wherein a program consists of functions called one after the other. It employs pointers for memory management, and thus, it is so much more complex but hugely powerful.
Java: Java syntax is more verbose in style and object-based, i.e., all things in Java are objects. Java supports encapsulation, inheritance, and polymorphism—the very essentials of OOP. Java never uses pointers; thus, it is more secure to use and handle.
b) Memory Management
C: C provides manual memory management using malloc and free, where the programmers have complete control over memory allocation and deallocation. It results in memory leaks and segmentation faults unless carried out with caution.
Java: Java automates memory management by garbage collection, which releases memory occupied by objects that are no longer being used occasionally. This is more convenient for developers because it eliminates memory leaks that would result from manual memory mismanagement.
c) Execution
C: C is a compiled language since the program is run with the assistance of the compiler and is translated into machine code directly, which is executable by the hardware of the respective system.
This renders the C programs very fast and efficient.
Java: Java is platform-independent since the code itself is initially compiled into bytecode and subsequently executed by the Java Virtual Machine (JVM). While this is an additional step of execution, it enables Java programs to execute on any computer with a JVM installed, and Java is highly portable.
d) Platform Dependency
C: C is platform-specific since the generated machine code during compilation is specific to the platform architecture (e.g., x86, ARM).
Java:
Java is cross-platform due to the JVM. Java code is compiled into bytecode, which is executable on any machine with a JVM, and therefore, Java programs are cross-platform.
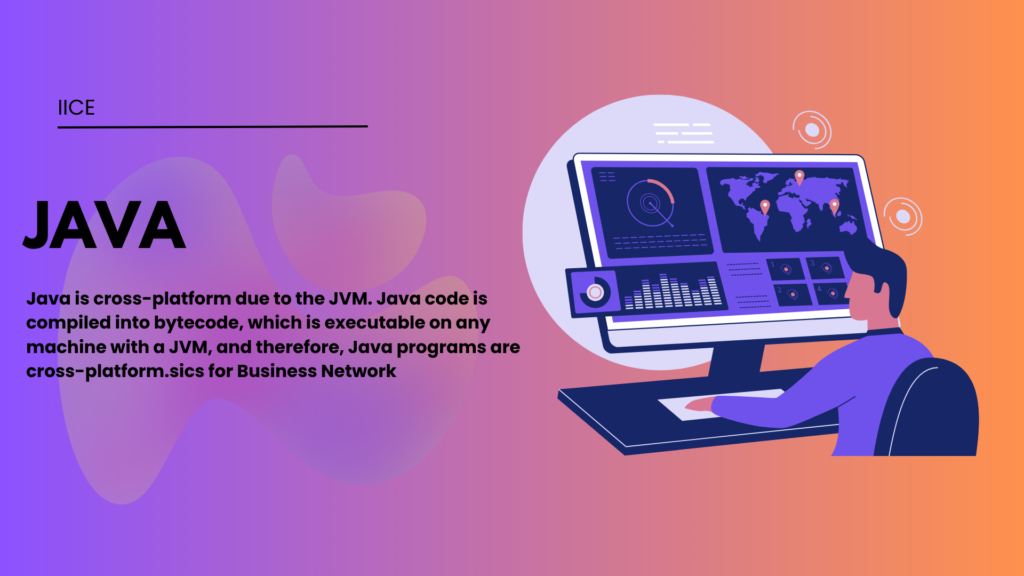
C: C is high-performance because it compiles directly to machine code. Hence, it is used in system programming, embedded systems, and software where maximum speed and efficiency are needed.
Java: Although Java is slower than C because of JVM overhead, optimizations such as Just-In-Time compilation have made Java performance comparable to most programs with average requirements.
f) Error Handling
C: C handles errors through error codes and explicit error checking, and thereby, error management is a bit complicated with a likelihood of human errors.
Java: Java handles exceptions through try-catch blocks, and hence, error handling becomes less complicated and code more maintainable and fault-tolerant.
g) Use Cases
C: C is used extensively for system programming, i.e., operating systems, embedded systems, and high-speed processing applications (e.g., real-time applications or gaming engines
3. Course Syllabus: C and Java
Knowing the syllabus of courses in C and Java will assist you in determining which language is most appropriate for your career goals. Here is a rough idea of what you will be studying in both:
C Programming Course Syllabus
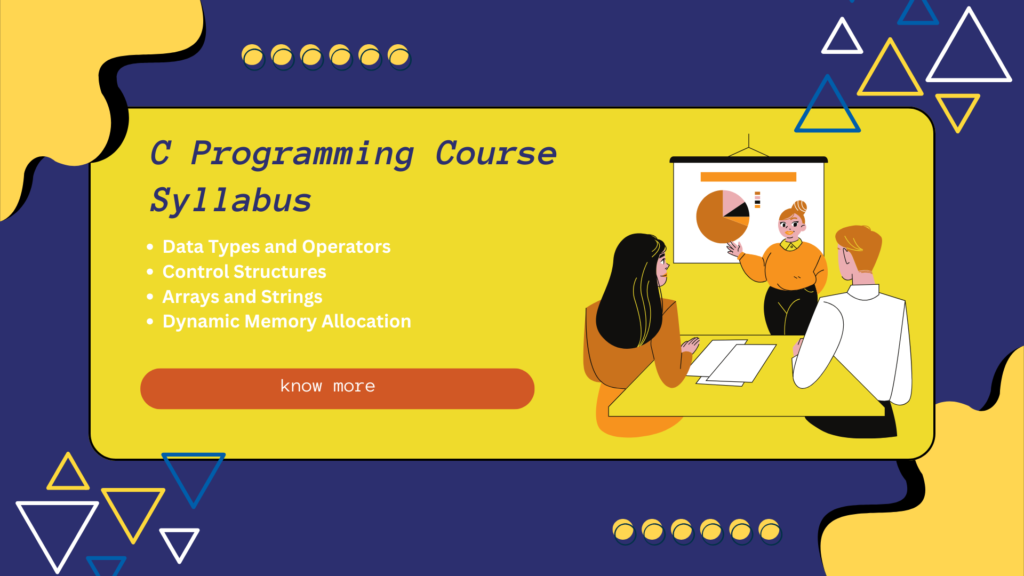
Data Types and Operators: Introduction to primitive data types, operators, and expressions.
Control Structures: Loops (for loop, while loop, do-while loop), conditional statements (if, switch).
Functions: Introduction to functions, function prototype, recursion.
Arrays and Strings: Array manipulating procedures, multi-dimensional arrays, string manipulating procedures.
Pointers: Concept of pointers, pointer operations, memory allocation using malloc and free.
Structures and Unions: Utilization of structures and unions to bundle data.
File Handling: File input/output operations and utilization of file pointers.
Dynamic Memory Allocation: Dynamic memory allocation with malloc, calloc, realloc, and free.
Advanced Topics: Linked list, stack, and queue implementation, sorting algorithms.
Java Programming Course Syllabus
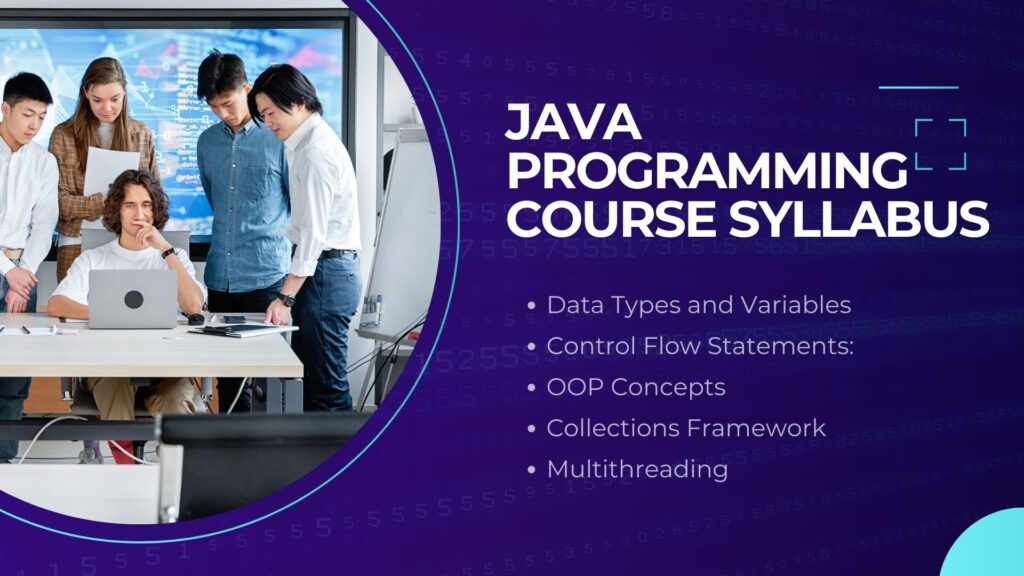
Data Types and Variables: Primitive data types, type casting, and variable declaration.
Control Flow Statements: Loops (for, while, do-while), if-else statements.
OOP Concepts: Classes, objects, constructors, inheritance, polymorphism, and abstraction.
Methods and Arrays: Method definitions, passing parameters to methods, method return values, and array manipulation.
Exception Handling: try-catch block, throwing exception, and user-defined exceptions.
String Handling: Working with the String class, regular expressions, and string manipulation.
Collections Framework: Learning Lists, Sets, Maps, and Iterators.
4. Career Prospects for Java and C Developers
C and Java both have great career prospects, but the fields and the positions can be different.
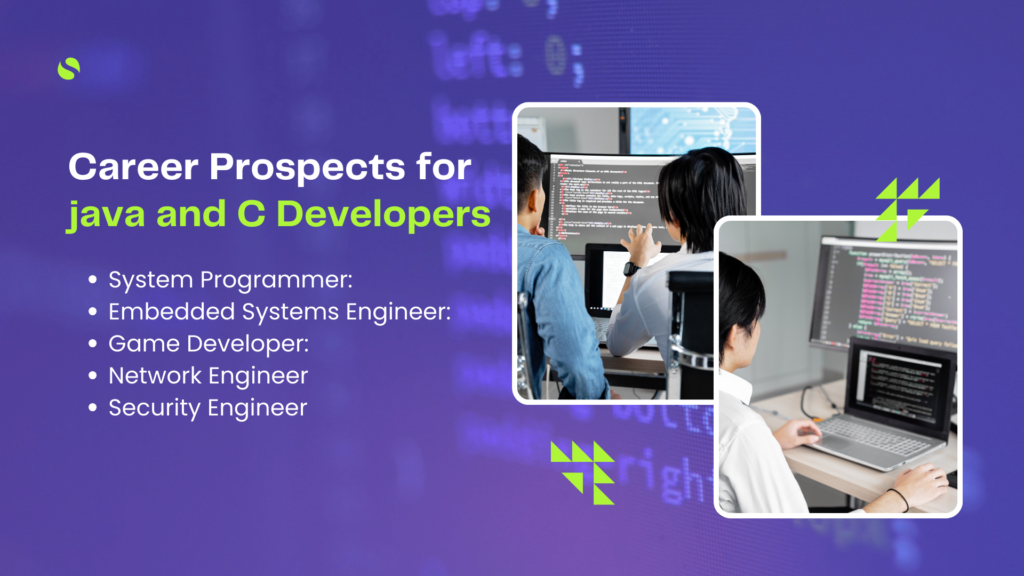
Job Prospects for C Developers
System Programmer: Writing and keeping low-level system software like operating systems, device drivers, and firmware.
Embedded Systems Engineer: I write hardware-related software like microcontrollers or IoT device software.
Game Developer: Creating high-performance games, particularly for game engines that need low-level access to memory and rapid execution.
Network Engineer: Building network software, protocol stacks, and high-speed networked applications.
Security Engineer: Designing and testing security, encryption, and data protection software.
Career Opportunities for Java Developers
Software Developer: Creating enterprise-sized applications, web applications, and mobile applications.
Android Developer: Creating Android mobile apps with Java help.
Web Developer: Creating server-side applications using Java frameworks such as Spring, Hibernate, and JavaServer Pages (JSP).
Enterprise Architect: Creating and sustaining large systems, particularly within companies using Java-based technology stacks.
Data Scientist: Java also finds applications for data processing within big data programs, and occupations such as Data Engineer necessitate familiarity with Java.
Conclusion
Both C and Java are strong programming languages with strengths based on their use. C is best for low-level programming and system-level programming, whereas Java is best for enterprise applications, web development, and mobile application development. Learning the differences between the two will enable you to pick the best one based on your career aspirations.
No matter what language you learn, C or Java, both are a good beginning for an efficient coder with numerous career opportunities ranging from embedded systems to enterprise software.
1. Which language is easier to learn, C or Java?
Java is also simpler to learn for new users since it is object-oriented and automatically manages memory (garbage collection). The syntax of Java is more structured and less error-prone compared to C.
C, although powerful, requires a stronger understanding of low-level concepts like memory management, pointers, and direct manipulation of the hardware. Such matters can render C and java more challenging for beginners.
2. What are the advantages of learning C over Java?
C and java offers more hardware and memory control and is hence appropriate for system programming, embedded systems, and high-performance applications.
It is faster than Java since C is converted into machine code directly, and therefore there is greater speed in execution.
3. Can I use C for mobile app development?
Even though C is not typically used to build typical mobile applications, it can be utilized for low-level operations or even to build games.
4. Are job opportunities better in C and Java?
Java offers a greater range of career opportunities, particularly in enterprise software, web applications, Android mobile app development, and big data systems. C jobs tend to be more specialized, including system programming, embedded systems, network programming, and game development positions.
5. Which one should I learn first, C or Java?
If you want to be a learn c and java system programmer, embedded system designer, or even a game developer, learning C is perhaps the way to go as it gives a precise view of low-level programming and memory management.